This blog post explores the top 20 Node.js coding interview questions along with their answers. It covers core concepts, modules, event-driven programming, and error handling. By familiarizing yourself with these…
Using Multiple EF Core DbContexts In a Single Application
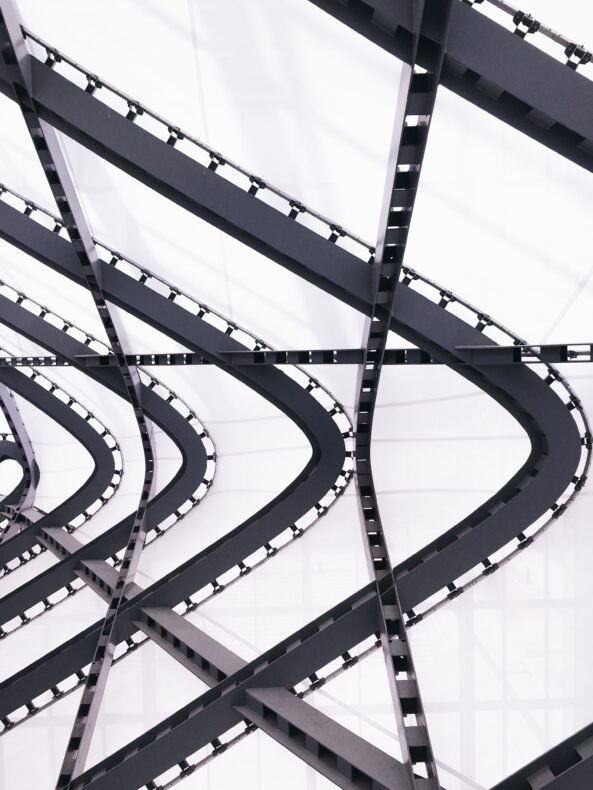
Introduction
In modern application development, it is common to work with multiple databases or data sources within a single application. Entity Framework Core (EF Core) is a popular Object-Relational Mapping (ORM) framework that simplifies data access in .NET applications. In this blog post, we will explore how to use multiple EF Core DbContexts in a single application.

Why Use Multiple DbContexts?
There are several scenarios where using multiple DbContexts becomes necessary:
- Working with multiple databases: When an application needs to interact with multiple databases, each database can be represented by a separate DbContext.
- Isolating concerns: By separating different parts of the application into separate DbContexts, it becomes easier to manage and maintain the codebase.
- Legacy databases: In some cases, an application may need to work with legacy databases that have different schemas or conventions. Using multiple DbContexts allows developers to handle these differences more effectively.
Implementing Multiple DbContexts
Let’s look at a step-by-step guide on how to implement multiple DbContexts in a single application:
Step 1: Define DbContext Classes
Create separate DbContext classes for each database or data source you want to work with. Each DbContext should inherit from the base DbContext class provided by EF Core.
public class AppDbContext : DbContext
{
// DbContext implementation for the main application database
}
public class CustomerDbContext : DbContext
{
// DbContext implementation for the customer database
}
public class ProductDbContext : DbContext
{
// DbContext implementation for the product database
}
Step 2: Configure Connection Strings

Configure the connection strings for each DbContext in the application’s configuration file (e.g., appsettings.json or web.config). Make sure to provide unique connection strings for each database.
"ConnectionStrings": {
"AppDbContext": "YourAppDbConnectionString",
"CustomerDbContext": "YourCustomerDbConnectionString",
"ProductDbContext": "YourProductDbConnectionString"
}
Step 3: Register DbContexts
In the application’s startup code, register each DbContext with the dependency injection container. This allows the DbContexts to be easily injected into other parts of the application.
services.AddDbContext<AppDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("AppDbContext")));
services.AddDbContext<CustomerDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("CustomerDbContext")));
services.AddDbContext<ProductDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("ProductDbContext")));
Step 4: Use Multiple DbContexts
Now that the DbContexts are registered, you can use them in different parts of your application. Simply inject the desired DbContext into the classes or services that need to access the corresponding database.
public class CustomerService
{
private readonly CustomerDbContext _customerDbContext;
public CustomerService(CustomerDbContext customerDbContext)
{
_customerDbContext = customerDbContext;
}
public List<Customer> GetCustomers()
{
return _customerDbContext.Customers.ToList();
}
}
public class ProductService
{
private readonly ProductDbContext _productDbContext;
public ProductService(ProductDbContext productDbContext)
{
_productDbContext = productDbContext;
}
public List<Product> GetProducts()
{
return _productDbContext.Products.ToList();
}
}
Conclusion
Using multiple EF Core DbContexts in a single application allows developers to work with multiple databases or data sources efficiently. By following the steps outlined in this blog post, you can easily implement and manage multiple DbContexts in your .NET application. This flexibility enables you to handle complex data scenarios and maintain a clean and modular codebase.

Remember to carefully design your DbContexts to ensure proper separation of concerns and avoid unnecessary complexity. With the right approach, leveraging multiple DbContexts can greatly enhance the scalability and maintainability of your application.