Hadoop Developer Course will help you to comprehend MapReduce Programming, how to set up an environment for the same, how to submit and execute MapReduce applications. We will begin from…
Top 30+ Java Interview Questions to Prepare In 2023

Java Interview Questions
Java is used everywhere! This is the reason why Java Certification is the most in-demand certification in the programming domain.Let us start by taking a look at some of the most frequently asked Java interview questions, Want to up-skill yourself to get ahead in Career? Check out the Top Trending Technologies.We have compiled a list of top Java interview questions which are classified into 7 sections.
Java Interview Questions and Answers
So let’s get started with the first set of basic Java Interview Questions.
Basic Java Interview Questions for Freshers
Q1. Explain public static void main(String args[]) in Java.
main() in Java is the entry point for any Java program. It is always written as public static void main(String[] args).
- public: Public is an access modifier, which is used to specify who can access this method. Public means that this Method will be accessible by any Class.
- static: It is a keyword in java which identifies it is class-based. main() is made static in Java so that it can be accessed without creating the instance of a Class. In case, main is not made static then the compiler will throw an error as main() is called by the JVM before any objects are made and only static methods can be directly invoked via the class.
- void: It is the return type of the method. Void defines the method which will not return any value.
- main: It is the name of the method which is searched by JVM as a starting point for an application with a particular signature only. It is the method where the main execution occurs.
- String args[]: It is the parameter passed to the main method.
Q2. Why Java is platform independent?
Java is called platform independent because of its byte codes which can run on any system irrespective of its underlying operating system.
Q3. Why Java is not 100% Object-oriented?
Java is not 100% Object-oriented because it makes use of eight primitive data types such as boolean, byte, char, int, float, double, long, short which are not objects.
Q4. What are wrapper classes in Java?
Wrapper classes convert the Java primitives into the reference types (objects). Every primitive data type has a class dedicated to it.
These are known as wrapper classes because they “wrap” the primitive data type into an object of that class. Refer to the below image which displays different primitive type, wrapper class and constructor argument.
Q5. What are constructors in Java?
In Java, constructor refers to a block of code which is used to initialize an object. It must have the same name as that of the class. Also, it has no return type and it is automatically called when an object is created.
There are two types of constructors:
- Default Constructor: In Java, a default constructor is the one which does not take any inputs. In other words, default constructors are the no argument constructors which will be created by default in case you no other constructor is defined by the user. Its main purpose is to initialize the instance variables with the default values. Also, it is majorly used for object creation.
- Parameterized Constructor: The parameterized constructor in Java, is the constructor which is capable of initializing the instance variables with the provided values. In other words, the constructors which take the arguments are called parameterized constructors.
Q6. What is singleton class in Java and how can we make a class singleton?
Singleton class is a class whose only one instance can be created at any given time, in one JVM. A class can be made singleton by making its constructor private.
Q7. What is the difference between equals() and == in Java?
Equals() method is defined in Object class in Java and used for checking equality of two objects defined by business logic.
“==” or equality operator in Java is a binary operator provided by Java programming language and used to compare primitives and objects.
public boolean equals(Object o) is the method provided by the Object class. The default implementation uses == operator to compare two objects.
For example: method can be overridden like String class. equals() method is used to compare the values of two objects.
Q8. When can you use the super keyword?
In Java, the super keyword is a reference variable that refers to an immediate parent class object.Java Certification Training CourseInstructor-led SessionsReal-life Case StudiesAssignmentsLifetime Access
When you create a subclass instance, you’re also creating an instance of the parent class, which is referenced to by the super reference variable.
The uses of the Java super Keyword are-
- To refer to an immediate parent class instance variable, use super.
- The keyword super can be used to call the method of an immediate parent class.
- Super() can be used to call the constructor of the immediate parent class.
Q9. What is the importance of reflection in Java?
Reflection is a runtime API for inspecting and changing the behavior of methods, classes, and interfaces. Java Reflection is a powerful tool that can be really beneficial.
Java Reflection allows you to analyze classes, interfaces, fields, and methods during runtime without knowing what they are called at compile time.
Reflection can also be used to create new objects, call methods, and get/set field values. External, user-defined classes can be used by creating instances of extensibility objects with their fully-qualified names.
Debuggers can also use reflection to examine private members of classes.
Q10. How to not allow serialization of attributes of a class in Java?
The NonSerialized attribute can be used to prevent member variables from being serialized.
You should also make an object that potentially contains security-sensitive data nonserializable if possible.
Apply the NonSerialized attribute to certain fields that store sensitive data if the object must be serialized. If you don’t exclude these fields from serialisation, the data they store will be visible to any programmes with serialization permission.
Q11. Can you call a constructor of a class inside another constructor?
Yes, we can call a constructor of a class inside another constructor. This is also called as constructor chaining. Constructor chaining can be done in 2 ways-
- Within the same class: For constructors in the same class, the this() keyword can be used.
- From the base class: The super() keyword is used to call the constructor from the base class.
The constructor chaining follows the process of inheritance. The constructor of the sub class first calls the constructor of the super class. - Due to this, the creation of sub class’s object starts with the initialization of the data members of the super class. The constructor chaining works similarly with any number of classes. Every constructor keeps calling the chain till the top of the chain.
Q12. Contiguous memory locations are usually used for storing actual values in an array but not in ArrayList. Explain.
An array generally contains elements of the primitive data types such as int, float, etc. In such cases, the array directly stores these elements at contiguous memory locations.
While an ArrayList does not contain primitive data types. An arrayList contains the reference of the objects at different memory locations instead of the object itself. That is why the objects are not stored at contiguous memory locations.
Q13. How is the creation of a String using new() different from that of a literal?
When we create a string using new(), a new object is created. Whereas, if we create a string using the string literal syntax, it may return an already existing object with the same name.
Q14. Why is synchronization necessary? Explain with the help of a relevant example.
Java allows multiple threads to execute. They may be accessing the same variable or object. Synchronization helps to execute threads one after another.
It is important as it helps to execute all concurrent threads while being in sync. It prevents memory consistency errors due to access to shared memory. An example of synchronization code is-
Programming & Frameworks Training
As we have synchronized this function, this thread can only use the object after the previous thread has used it.
Q15. Explain the term “Double Brace Initialization” in Java?
Double Brace Initialization is a Java term that refers to the combination of two independent processes. There are two braces used in this.

The first brace creates an anonymous inner class. The second brace is an initialization block. When these both are used together, it is known as Double Brace Initialization. The inner class has a reference to the enclosing outer class, generally using the ‘this’ pointer.
It is used to do both creation and initialization in a single statement. It is generally used to initialize collections. It reduces the code and also makes it more readable.
Q16. Why is it said that the length() method of String class doesn’t return accurate results?
The length() method of String class doesn’t return accurate results because
it simply takes into account the number of characters within in the String. In other words, code points outside of the BMP (Basic Multilingual Plane), that is, code points having a value of U+10000 or above, will be ignored.
The reason for this is historical. One of Java’s original goals was to consider all text as Unicode; yet, Unicode did not define code points outside of the BMP at the time. It was too late to modify char by the time Unicode specified such code points.
Q17. What is a package in Java? List down various advantages of packages.
Packages in Java, are the collection of related classes and interfaces which are bundled together.
By using packages, developers can easily modularize the code and optimize its reuse. Also, the code within the packages can be imported by other classes and reused. Below I have listed down a few of its advantages:
- Packages help in avoiding name clashes
- They provide easier access control on the code
- Packages can also contain hidden classes which are not visible to the outer classes and only used within the package
- Creates a proper hierarchical structure which makes it easier to locate the related classes
Q18. Why pointers are not used in Java?
Java doesn’t use pointers because they are unsafe and increases the complexity of the program. Since, Java is known for its simplicity of code, adding the concept of pointers will be contradicting.
Moreover, since JVM is responsible for implicit memory allocation, thus in order to avoid direct access to memory by the user, pointers are discouraged in Java.
Q19. What is JIT compiler in Java?
JIT stands for Just-In-Time compiler in Java. It is a program that helps in converting the Java bytecode into instructions that are sent directly to the processor.
By default, the JIT compiler is enabled in Java and is activated whenever a Java method is invoked. The JIT compiler then compiles the bytecode of the invoked method into native machine code, compiling it “just in time” to execute.
Once the method has been compiled, the JVM summons the compiled code of that method directly rather than interpreting it. This is why it is often responsible for the performance optimization of Java applications at the run time.
Q20. Define a Java Class.
A class in Java is a blueprint which includes all your data. A class contains fields (variables) and methods to describe the behavior of an object. Let’s have a look at the syntax of a class.
123 | class Abc { member variables // class body methods} |
Q21. What is an object in Java and how is it created?
An object is a real-world entity that has a state and behavior. An object has three characteristics:
- State
- Behavior
- Identity
An object is created using the ‘new’ keyword. For example:
ClassName obj = new ClassName();
Q22. What is Object Oriented Programming?
Object-oriented programming or popularly known as OOPs is a programming model or approach where the programs are organized around objects rather than logic and functions.
In other words, OOP mainly focuses on the objects that are required to be manipulated instead of logic. This approach is ideal for the programs large and complex codes and needs to be actively updated or maintained.
Q23. What are the main concepts of OOPs in Java?
Object-Oriented Programming or OOPs is a programming style that is associated with concepts like:
- Inheritance: Inheritance is a process where one class acquires the properties of another.
- Encapsulation: Encapsulation in Java is a mechanism of wrapping up the data and code together as a single unit.
- Abstraction: Abstraction is the methodology of hiding the implementation details from the user and only providing the functionality to the users.
- Polymorphism: Polymorphism is the ability of a variable, function or object to take multiple forms.

Comprehensive Java Course Certification Training
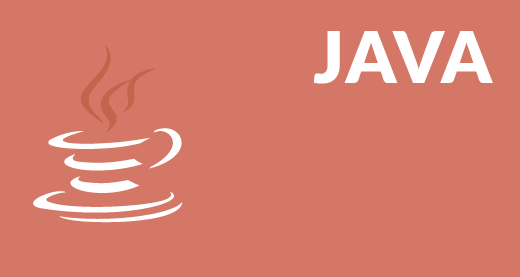
Please put your comments how did you like java interview questions and we will provide more interview practice questions.