This blog post explores the top 20 Node.js coding interview questions along with their answers. It covers core concepts, modules, event-driven programming, and error handling. By familiarizing yourself with these…
Modern OOP: Classes, Constructors, and Prototypal Inheritance

Learn about Object-Oriented Programming (OOP) concepts such as classes, constructors, and prototypal inheritance for JavaScript
Understand how these concepts contribute to building robust and maintainable code. Explore examples in JavaScript and Python.
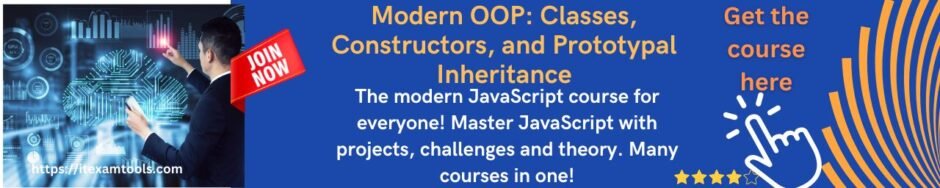
Start your journey in software development and leverage the power of OOP for creating scalable applications.
What is Object-Oriented Programming
In the world of programming, Object-Oriented Programming (OOP) has become a widely adopted paradigm due to its ability to organize and structure code in a more modular and reusable manner.
One of the key concepts in OOP is the use of classes, constructors, and prototypal inheritance.
In this blog post, we will explore these modern Object-Oriented Programming concepts and understand how they contribute to building robust and maintainable code.
Classes in Object-Oriented Programming : The Blueprint of Objects
In OOP, a class serves as a blueprint for creating objects. It defines the properties and behaviors that an object of that class will possess.
By defining a class, we can create multiple instances of that class, each with its own set of properties and behaviors.
Modern programming languages, such as JavaScript and Python, have introduced class syntax to make it easier to define and work with classes.
Using the class keyword, we can define a class and its properties and methods. Let’s take a look at an example:
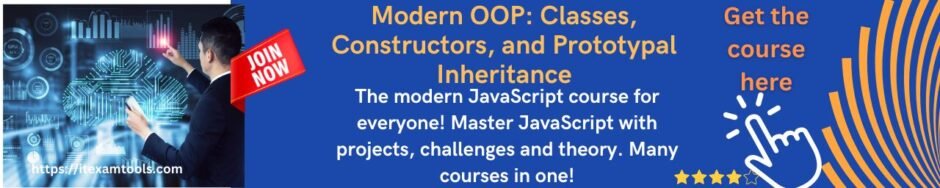
class Car { constructor(make, model, year) { this.make = make; this.model = model; this.year = year; } startEngine() { console.log("Engine started!"); } stopEngine() { console.log("Engine stopped!"); } } const myCar = new Car("Tesla", "Model 3", 2021);
In the above example, we define a Car class with properties like make, model, and year. We also define methods like startEngine() and stopEngine().
We can then create an instance of the Car class using the new keyword and assign it to the variable myCar.
Constructors in Object-Oriented Programming : Initializing Objects
Constructors are special methods within a class that are automatically called when an object is created.
They are responsible for initializing the object’s properties and performing any necessary setup.
In the previous example, the constructor function within the Car class is used to initialize the make, model, and year properties of the car object.
Constructors allow us to provide initial values for the object’s properties and ensure that the object is in a valid state when it is created.
They play a crucial role in setting up the object’s initial state and preparing it for use.
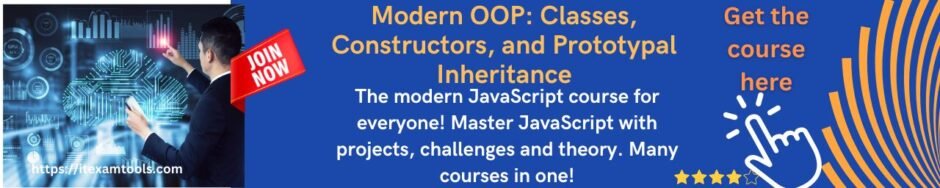
Prototypal Inheritance: Building Relationships
Prototypal inheritance is a fundamental concept in Object-Oriented Programming that allows objects to inherit properties and methods from other objects.
It enables code reuse and the creation of hierarchical relationships between objects.
In JavaScript, prototypal inheritance is achieved through the prototype chain. Each object in JavaScript has a prototype property that points to another object.
When a property or method is accessed on an object, JavaScript first checks if the object itself has that property or method.
If not, it looks up the prototype chain until it finds the property or method.
Let’s see an example to understand how prototypal inheritance works:
class Animal { constructor(name) { this.name = name; } speak() { console.log(this.name + " makes a sound."); } } class Dog extends Animal { constructor(name, breed) { super(name); this.breed = breed; } bark() { console.log(this.name + " barks!"); } } const myDog = new Dog("Buddy", "Labrador"); myDog.speak(); myDog.bark();
In the above example, we define an Animal class with a speak() method. We then create a Dog class that extends the Animal class using the extends keyword.
The Dog class has its own constructor and a bark() method.
By using the super keyword in the Dog class’s constructor, we call the constructor of the parent class to initialize the name property.
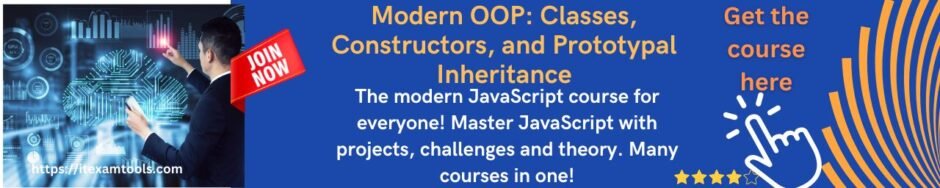
This is an example of inheritance, where the Dog class inherits the properties and methods of the Animal class.
By leveraging prototypal inheritance, we can create a hierarchy of classes and reuse common functionality across different objects. This leads to more efficient and organized code.
Conclusion
Classes, constructors, and prototypal inheritance are essential concepts in modern OOP.
They provide a structured and modular approach to building software systems.
By understanding and applying these concepts, developers can create code that is easier to maintain, extend, and debug.
As you continue your journey in software development, take the time to explore and experiment with these concepts. Embrace the power of OOP and leverage the benefits it offers in creating robust and scalable applications.
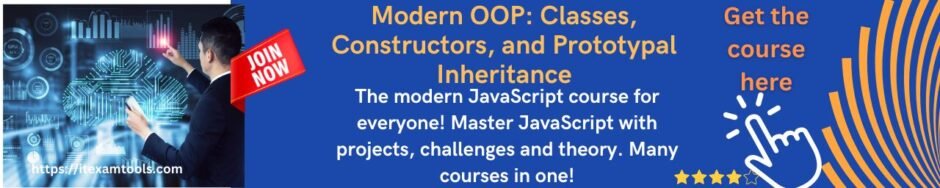
https://itexamsusa.blogspot.com/2023/12/mastering-matlab-programming-for.html
https://itexamsusa.blogspot.com/2023/12/monolith-vs-microservices-which-one-is.html
https://itexamsusa.blogspot.com/2023/12/publicprivate-keypairs-and-generating.html
https://itexamsusa.blogspot.com/2023/10/exam-dp-203-data-engineering-on.html
https://itexamsusa.blogspot.com/2023/10/ccnp-enterprise-advanced-routing-enarsi.html
https://itexamsusa.blogspot.com/2023/10/red-hat-certified-engineerrhce-ex294.html
https://itexamsusa.blogspot.com/2023/09/github-actions-to-auto-build-your.html