Encryption and Decryption in Python: A Comprehensive Guide
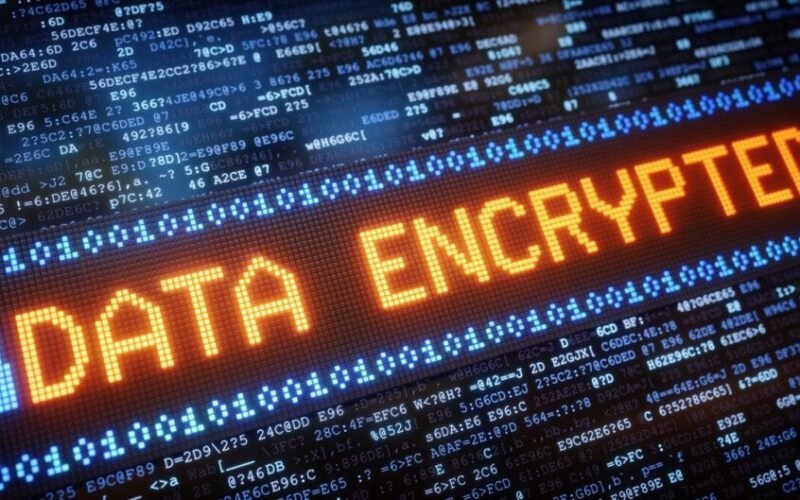
Learn how to implement encryption and decryption algorithms in Python.
Understand the different types of encryption, such as symmetric and asymmetric encryption. Explore the cryptography
and pycryptodome
libraries for implementing encryption and decryption.
Follow best practices for encryption and decryption, including using strong encryption algorithms, protecting your keys, implementing key rotation, using secure communication channels, and regularly testing and auditing your implementation.
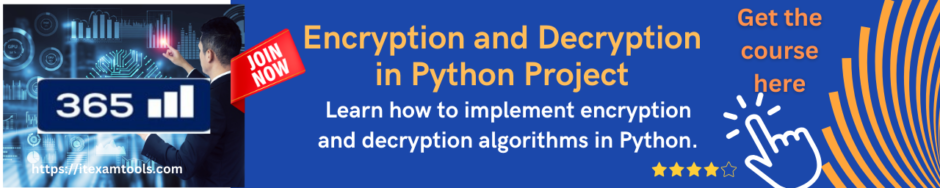
Ensure the security and integrity of your data with encryption and decryption techniques.
Introduction
Encryption and decryption are fundamental concepts in the field of computer science and data security. In this blog post, we will explore how to implement encryption and decryption algorithms in Python.
Understanding Encryption
Encryption is the process of converting plain text into a secret code, known as cipher text, to protect sensitive information from unauthorized access.
It ensures that even if an attacker gains access to the cipher text, they cannot understand its contents without the decryption key.
Types of Encryption
There are two main types of encryption: symmetric encryption and asymmetric encryption.
Symmetric Encryption
Symmetric encryption, also known as secret key encryption, uses the same key for both encryption and decryption.
The key must be kept secret and shared securely between the sender and the receiver.
Examples of symmetric encryption algorithms include Advanced Encryption Standard (AES) and Data Encryption Standard (DES).
Asymmetric Encryption
Asymmetric encryption, also known as public key encryption, uses a pair of keys: a public key for encryption and a private key for decryption.
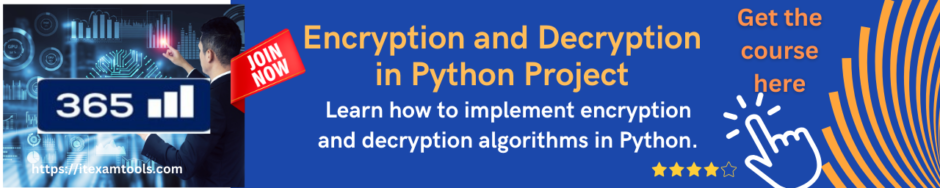
The public key can be freely distributed, while the private key must be kept secret. Examples of asymmetric encryption algorithms include RSA and Elliptic Curve Cryptography (ECC).
Implementing Encryption and Decryption in Python
Python provides several libraries and modules that make it easy to implement encryption and decryption algorithms. Let’s explore some of them:
1. The `cryptography` Library
The `cryptography` library is a popular choice for implementing encryption and decryption in Python. It provides a high-level interface for various cryptographic operations.
Here’s an example of how to use the `cryptography` library to encrypt and decrypt data:
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
# Create a Fernet cipher object
cipher = Fernet(key)
# Encrypt data
encrypted_data = cipher.encrypt(b"Hello, World!")
# Decrypt data
decrypted_data = cipher.decrypt(encrypted_data)
print(decrypted_data.decode())
2. The `pycryptodome` Library
The `pycryptodome` library is another powerful library for implementing encryption and decryption in Python.
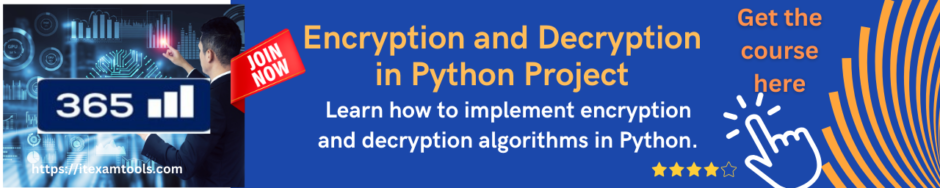
It supports a wide range of cryptographic algorithms and provides low-level access to cryptographic primitives. Here’s an example of how to use the `pycryptodome` library to encrypt and decrypt data:
from Crypto.Cipher import AES
# Generate a key
key = b"supersecretkey123"
# Create an AES cipher object
cipher = AES.new(key, AES.MODE_ECB)
# Encrypt data
encrypted_data = cipher.encrypt(b"Hello, World!")
# Decrypt data
decrypted_data = cipher.decrypt(encrypted_data)
print(decrypted_data.decode())
Best Practices for Encryption and Decryption
When implementing encryption and decryption in Python, it’s important to follow best practices to ensure the security of your data:
1. Use Strong Encryption Algorithms
Choose encryption algorithms that are widely accepted and considered secure. Avoid using outdated or weak algorithms that are vulnerable to attacks.
2. Protect Your Keys
Keep your encryption keys secure and separate from the encrypted data. Use strong passwords or key management systems to protect your keys.
3. Implement Key Rotation
Regularly rotate your encryption keys to minimize the impact of a potential key compromise. This ensures that even if a key is compromised, the attacker will have limited access to the encrypted data.
4. Use Secure Communication Channels
When transmitting encrypted data, ensure that you use secure communication channels, such as HTTPS, to prevent eavesdropping or man-in-the-middle attacks.
5. Test and Audit Your Implementation
Regularly test your encryption and decryption implementation for vulnerabilities. Conduct security audits to identify any weaknesses and address them promptly.
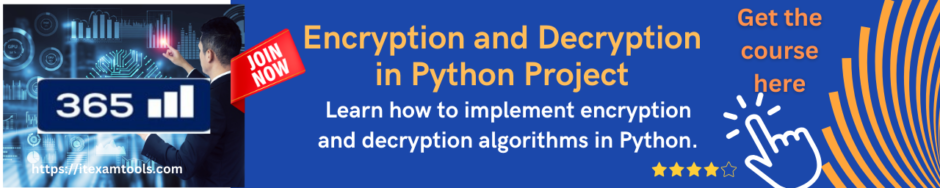
Conclusion
Encryption and decryption are essential techniques for protecting sensitive information in today’s digital world.
In this blog post, we explored the concepts of encryption and decryption, discussed different types of encryption algorithms, and learned how to implement encryption and decryption in Python using the `cryptography` and `pycryptodome` libraries.
By following best practices and staying updated on the latest advancements in encryption technology, you can ensure the security and integrity of your data.
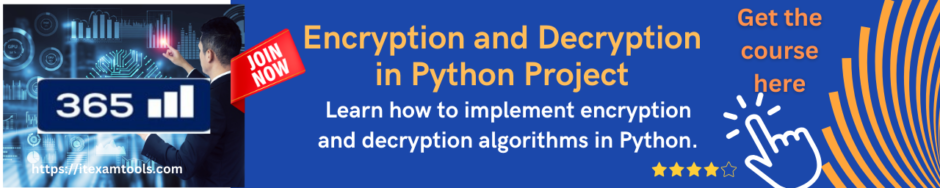
https://itexamsusa.blogspot.com/p/ms-721-exam-q.html
https://itexamsusa.blogspot.com/p/cism.html
https://itexamsusa.blogspot.com/p/ccna.html
https://itexamsusa.blogspot.com/p/free-it-exams-matgerials.html
https://itexamsusa.blogspot.com/p/az-800-exam.html
https://itexamsusa.blogspot.com/p/ms-721-exam-q.html
https://itexamsusa.blogspot.com/2023/10/ccnp-enterprise-advanced-routing-enarsi.html
https://itexamsusa.blogspot.com/2023/12/publicprivate-keypairs-and-generating.html
https://itexamtools.com/ethical-hacking-and-penetration-tools-for-fair-use/