Introduction In the modern era of cloud computing, software architecture plays a crucial role in enabling organizations to leverage the full potential of the cloud. With the increasing adoption of…
Integrating Different Services in Your Serverless Projects: A Comprehensive Guide
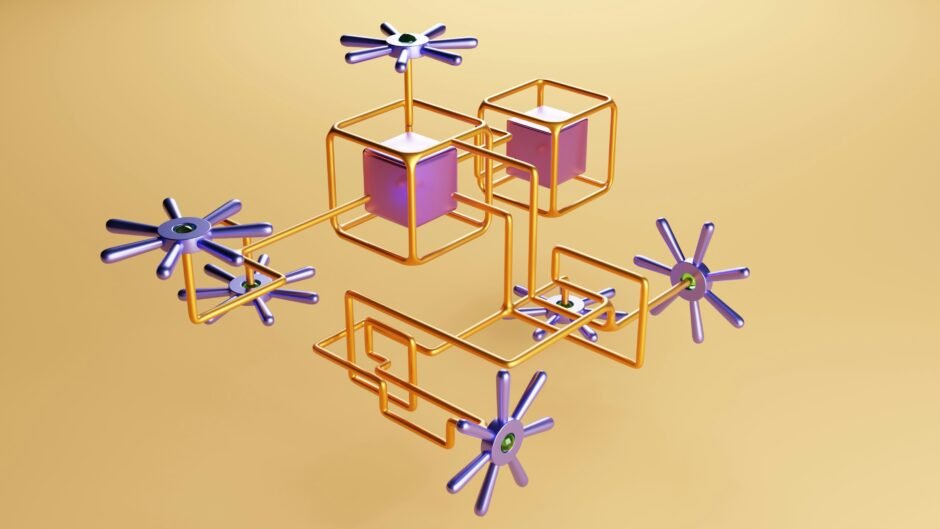
Learn how to integrate services like S3, Kinesis, SNS, and SQS in your serverless architecture.
This blog post provides examples in Python, Node.js, Java, and Ruby for integrating these services.
Discover the advantages of serverless architecture and how it can enhance the functionality and flexibility of your applications.
Get started with integrating these services in your own serverless projects today.
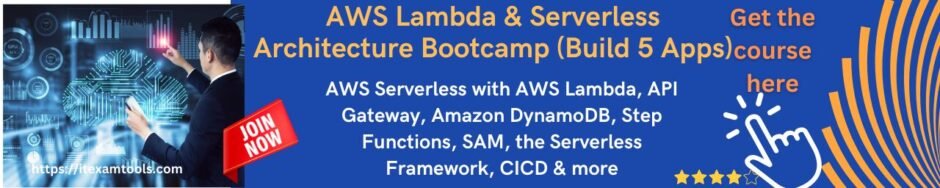
Introduction
Serverless architecture has become increasingly popular in recent years due to its scalability, cost-effectiveness, and ease of deployment.
One of the key advantages of serverless is the ability to integrate various services seamlessly.
In this blog post, we will explore how to integrate services like S3, Kinesis, SNS, SQS, and more in your serverless projects.
1. Integrating S3 to serverless architecture
S3, or Simple Storage Service, is a highly scalable object storage service provided by AWS. It is commonly used to store and retrieve large amounts of data.
To integrate S3 in your serverless project, you can use the AWS SDK for your preferred programming language. Here’s an example in Python:
import boto3
def upload_file_to_s3(file_path, bucket_name):
s3 = boto3.client('s3')
s3.upload_file(file_path, bucket_name, file_path.split('/')[-1])
2. Integrating Kinesis
AWS Kinesis is a fully managed streaming service that allows you to collect, process, and analyze real-time data.
To integrate Kinesis in your serverless project, you can use the Kinesis SDK provided by AWS. Here’s an example in Node.js:
const AWS = require('aws-sdk');
const kinesis = new AWS.Kinesis();
const putRecordToKinesis = async (streamName, data) => {
const params = {
StreamName: streamName,
Data: JSON.stringify(data),
PartitionKey: '1'
};
await kinesis.putRecord(params).promise();
};
3. Integrating SNS
AWS SNS, or Simple Notification Service, is a highly flexible and scalable messaging service. It enables you to send notifications to a variety of endpoints, such as email, SMS, or HTTP.
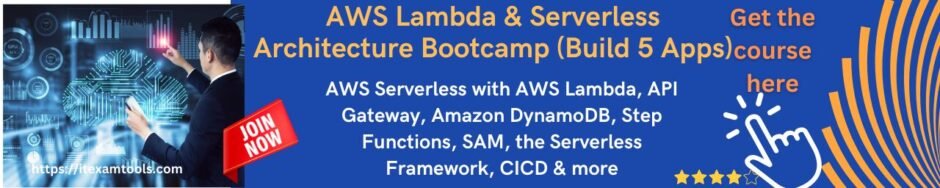
To integrate SNS in your serverless project, you can use the AWS SDK. Here’s an example in Java:
import com.amazonaws.services.sns.AmazonSNS;
import com.amazonaws.services.sns.AmazonSNSClientBuilder;
public class SnsIntegration {
public static void publishMessage(String topicArn, String message) {
AmazonSNS snsClient = AmazonSNSClientBuilder.defaultClient();
snsClient.publish(topicArn, message);
}
}
4. Integrating SQS
AWS SQS, or Simple Queue Service, is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications.
To integrate SQS in your serverless project, you can use the AWS SDK. Here’s an example in Ruby:
require 'aws-sdk-sqs'
sqs = Aws::SQS::Client.new(region: 'us-west-2')
def send_message_to_sqs(queue_url, message_body)
sqs.send_message({
queue_url: queue_url,
message_body: message_body
})
end
Conclusion
Integrating different services like S3, Kinesis, SNS, SQS, and more in your serverless projects can greatly enhance their functionality and flexibility.
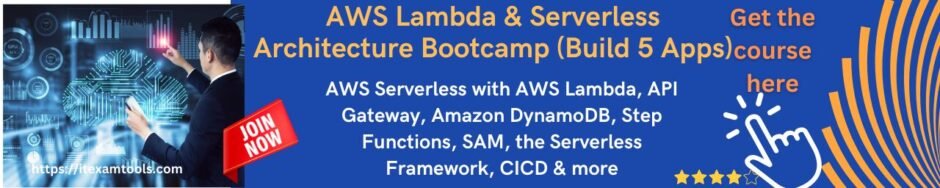
By leveraging the power of these services, you can build robust and scalable applications without the need to manage infrastructure.
We hope this guide has provided you with a solid foundation for integrating these services in your own serverless projects.
https://itexamsusa.blogspot.com/2023/12/mastering-matlab-programming-for.html
https://itexamsusa.blogspot.com/2023/12/monolith-vs-microservices-which-one-is.html
https://itexamsusa.blogspot.com/2023/12/publicprivate-keypairs-and-generating.html
https://itexamsusa.blogspot.com/2023/10/exam-dp-203-data-engineering-on.html
https://itexamsusa.blogspot.com/2023/10/ccnp-enterprise-advanced-routing-enarsi.html
https://itexamsusa.blogspot.com/2023/10/red-hat-certified-engineerrhce-ex294.html
https://itexamsusa.blogspot.com/2023/09/github-actions-to-auto-build-your.html