Cisco OSPF Basic Configuration In this Cisco CCNA tutorial, you’ll learn how to do a basic configuration of OSPF on our Cisco routers. Scroll down for the video and also text…
Ethereum Blockchain Developer Bootcamp With Solidity (2023)
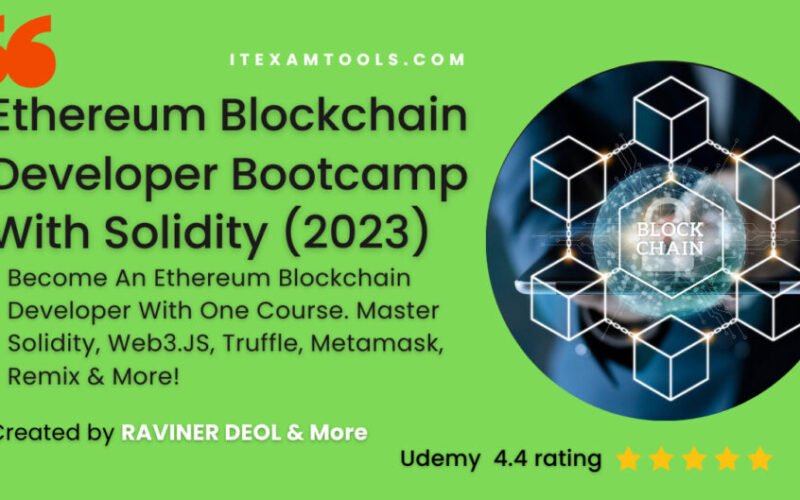
Become An Ethereum Blockchain Developer With One Course. Master Solidity, Web3.JS, Truffle, Metamask, Remix & More!
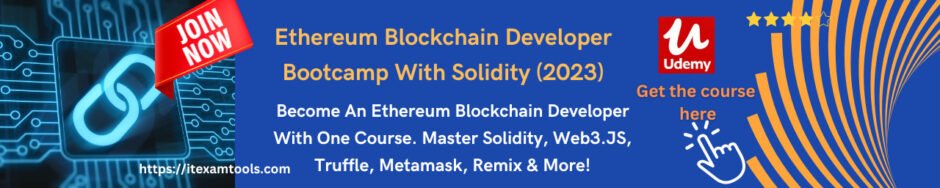
Ignite Your IT Career with the Ethereum Blockchain Developer Bootcamp
In the fast-paced world of IT, staying ahead of the curve is essential for unlocking your full potential. If you’re a tech enthusiast yearning to dive into the captivating realm of blockchain development, look no further than the Ethereum Blockchain Developer Bootcamp With Solidity (2023) on Udemy. This immersive and budget-friendly online course is your passport to mastering the art of blockchain and becoming a proficient Ethereum Blockchain Developer.
Embark on a Journey of Blockchain Mastery
1. Solidity Programming By Building Projects
The backbone of blockchain development lies in mastering Solidity, the programming language specifically designed for creating smart contracts on the Ethereum platform. Through hands-on projects, you’ll learn the ins and outs of Solidity, allowing you to craft secure, efficient, and robust smart contracts that power decentralized applications.
2. Blockchain Usage (Theory + Practice)
Delve into the theory and practice of blockchain technology, uncovering its foundational principles and real-world applications. From understanding the concepts of blocks, chains, and cryptography to exploring how blockchain disrupts industries like finance, supply chain, and more, you’ll gain a comprehensive perspective on this transformative technology.
3. Smart Contract Usage (Theory + Practice)
Smart contracts are the driving force behind decentralized applications, enabling automated and tamper-proof interactions. You’ll not only grasp the theory behind smart contracts but also dive into hands-on projects that allow you to create your own, opening doors to a realm of innovative possibilities.
4. Using Ethereum’s Core Development Tools
Navigate the Ethereum ecosystem with ease as you become adept at utilizing the core development tools. Whether it’s the Ethereum Wallet, Remix IDE, or the Ethereum CLI, you’ll gain the proficiency needed to efficiently develop, deploy, and manage your blockchain projects.
5. The Development Functions Of Ethereum
Explore the functions and capabilities of Ethereum, understanding its role as a decentralized platform for building and deploying applications. From executing transactions to deploying smart contracts and interacting with blockchain networks, you’ll harness the power of Ethereum’s development environment.
Unleash Your Potential as a Blockchain Developer
6. Truffle Development By Building Projects
Unravel the potential of Truffle, a popular development framework for Ethereum. By engaging in practical projects, you’ll harness Truffle’s features to streamline your development process, automate testing, and ensure the security and reliability of your blockchain applications.
7. How Decentralized Technology Works
Demystify the inner workings of decentralized technology, unveiling the intricacies of distributed ledgers, consensus algorithms, and the peer-to-peer nature of blockchain networks. You’ll emerge with a clear understanding of how decentralization empowers secure, transparent, and tamper-resistant systems.
8. The Purpose And Structure Of Solidity Code
Master the art of writing clean, efficient, and readable Solidity code. You’ll delve into the purpose behind various code structures, ensuring your smart contracts are not only functional but also maintainable and easily comprehensible by fellow developers.
Conclusion: Elevate Your IT Career with Blockchain Brilliance
The Ethereum Blockchain Developer Bootcamp With Solidity (2023) course isn’t just an ordinary online program; it’s your gateway to becoming a proficient Ethereum Blockchain Developer, a realm rich with potential and innovation. By enrolling in this transformative course, you’re investing in more than just your education – you’re investing in your future as a blockchain visionary.
Whether you’re an aspiring developer or a seasoned IT professional, this course equips you with the tools, knowledge, and practical experience needed to thrive in the captivating world of blockchain development. Embrace the opportunity to learn, innovate, and create as you take the leap into blockchain mastery. Enroll in the Ethereum Blockchain Developer Bootcamp With Solidity (2023) today and unleash your potential as a blockchain trailblazer. Your IT career will soar to new heights, powered by the transformative force of blockchain technology.

let’s incorporate practical code examples to illustrate the key concepts covered in the Ethereum Blockchain Developer Bootcamp With Solidity (2023) course:
1. Solidity Programming By Building Projects
solidityCopy code// Sample Solidity smart contract for a simple voting system
pragma solidity ^0.8.0;
contract Voting {
mapping (bytes32 => uint256) public votes;
function voteForCandidate(bytes32 candidate) public {
votes[candidate] += 1;
}
function getVotesForCandidate(bytes32 candidate) public view returns (uint256) {
return votes[candidate];
}
}
2. Blockchain Usage (Theory + Practice)
Blockchain technology ensures data integrity and immutability. Here’s a simple Python example using the hashlib
library to create a basic blockchain:
pythonCopy codeimport hashlib
class Block:
def __init__(self, data, previous_hash):
self.data = data
self.previous_hash = previous_hash
self.hash = self.calculate_hash()
def calculate_hash(self):
data = str(self.data).encode('utf-8')
return hashlib.sha256(data).hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
def create_genesis_block(self):
return Block("Genesis Block", "0")
def add_block(self, data):
previous_block = self.chain[-1]
new_block = Block(data, previous_block.hash)
self.chain.append(new_block)
# Create a blockchain and add blocks
blockchain = Blockchain()
blockchain.add_block("Transaction 1")
blockchain.add_block("Transaction 2")
3. Smart Contract Usage (Theory + Practice)
solidityCopy code// Sample Solidity smart contract for a crowdfunding campaign
pragma solidity ^0.8.0;
contract Crowdfunding {
address public creator;
uint256 public goal;
uint256 public raisedAmount = 0;
constructor(uint256 _goal) {
creator = msg.sender;
goal = _goal;
}
function contribute() public payable {
require(msg.value > 0, "Contribution must be greater than 0");
raisedAmount += msg.value;
}
function finalize() public {
require(msg.sender == creator, "Only the creator can finalize");
require(raisedAmount >= goal, "Goal not reached");
// Transfer funds to creator
payable(creator).transfer(address(this).balance);
}
}
4. Using Ethereum’s Core Development Tools
Here’s an example of using the Ethereum Remix IDE to deploy a simple smart contract:
- Open Remix IDE (https://remix.ethereum.org/).
- Create a new Solidity file and paste the smart contract code.
- Compile the contract using the “Solidity Compiler” tab.
- Deploy the contract using the “Deploy & Run Transactions” tab.
5. The Development Functions Of Ethereum
Interacting with Ethereum using Web3.js:
javascriptCopy codeconst Web3 = require('web3');
const web3 = new Web3('http://localhost:8545'); // Connect to a local Ethereum node
async function main() {
const accounts = await web3.eth.getAccounts();
console.log('Accounts:', accounts);
const balance = await web3.eth.getBalance(accounts[0]);
console.log('Balance:', web3.utils.fromWei(balance, 'ether'), 'ETH');
}
main();
6. Truffle Development By Building Projects
Sample Truffle project directory structure:
luaCopy codemy-dapp/
|-- contracts/
| |-- MyContract.sol
|
|-- migrations/
| |-- 1_initial_migration.js
|
|-- test/
| |-- myContract.test.js
|
|-- truffle-config.js
7. How Decentralized Technology Works
Understanding consensus algorithms like Proof of Work (PoW) and Proof of Stake (PoS). Sample PoW mining function in Python:
pythonCopy codeimport hashlib
def mine_block(previous_hash, transactions):
nonce = 0
while True:
data = f'{previous_hash}{transactions}{nonce}'.encode('utf-8')
hash_value = hashlib.sha256(data).hexdigest()
if hash_value[:4] == '0000':
return nonce, hash_value
nonce += 1
# Example usage
previous_hash = '0000abcd...'
transactions = 'Transaction data...'
nonce, block_hash = mine_block(previous_hash, transactions)
8. The Purpose And Structure Of Solidity Code
Understanding the structure of Solidity contracts:
solidityCopy codepragma solidity ^0.8.0;
contract MyContract {
// State variables
uint256 public myNumber;
// Constructor
constructor() {
myNumber = 42;
}
// Function to update the state variable
function setNumber(uint256 newValue) public {
myNumber = newValue;
}
// Function to retrieve the state variable
function getNumber() public view returns (uint256) {
return myNumber;
}
}
These practical code examples provide a glimpse into the hands-on experience and skills you’ll acquire through the Ethereum Blockchain Developer Bootcamp With Solidity (2023) course. By immersing yourself in real-world projects and diving deep into blockchain concepts, you’ll be well-equipped to embark on a successful career in blockchain development. Enroll in the course today to unlock a world of opportunities in the exciting realm of blockchain technology.

Here are 20 multiple-choice questions related to the topics covered in the Ethereum Blockchain Developer Bootcamp With Solidity (2023) course:
1. Solidity Programming By Building Projects
a) Which programming language is commonly used for creating smart contracts on the Ethereum platform?
- JavaScript
- Solidity
- Python
- Ruby
Answer: b) Solidity
2. Blockchain Usage (Theory + Practice)
a) What is the primary benefit of blockchain technology in ensuring data integrity?
- Faster data processing
- Centralized control
- Data immutability
- Data duplication
Answer: c) Data immutability
3. Smart Contract Usage (Theory + Practice)
a) What is a smart contract in the context of blockchain?
- A digital representation of a physical contract
- A program that executes on a blockchain network
- A document outlining the terms of a business agreement
- A chatbot for customer service
Answer: b) A program that executes on a blockchain network
4. Using Ethereum’s Core Development Tools
a) What is the purpose of the Ethereum Wallet?
- To store physical currency
- To mine cryptocurrency
- To deploy smart contracts
- To play online games
Answer: c) To deploy smart contracts
5. The Development Functions Of Ethereum
a) What is the role of an Ethereum account?
- It represents a physical wallet for storing cryptocurrency
- It stores data and programs on the blockchain
- It acts as a ledger for recording financial transactions
- It connects to social media platforms
Answer: b) It stores data and programs on the blockchain
6. Truffle Development By Building Projects
a) What is Truffle used for in Ethereum development?
- Writing emails
- Managing personal finances
- Developing smart contracts and decentralized applications
- Playing online games
Answer: c) Developing smart contracts and decentralized applications
7. How Decentralized Technology Works
a) Which consensus algorithm requires miners to solve complex mathematical puzzles to validate transactions?
- Proof of Work (PoW)
- Proof of Stake (PoS)
- Proof of Concept (PoC)
- Proof of Authority (PoA)
Answer: a) Proof of Work (PoW)
8. The Purpose And Structure Of Solidity Code
a) What is the primary purpose of a Solidity smart contract?
- To create artistic designs
- To manage user accounts
- To deploy web applications
- To define and automate business logic on the blockchain
Answer: d) To define and automate business logic on the blockchain
9. What programming language is primarily used for creating smart contracts on the Ethereum platform? a) JavaScript b) Python c) Solidity d) C++
Answer: c) Solidity
10. What key feature ensures data immutability in a blockchain? a) Centralized control b) Decentralization c) Data duplication d) Cryptographic hashing
makefileCopy code**Answer: d) Cryptographic hashing**
11. Which term refers to a self-executing contract with the terms of the agreement directly written into code? a) Blockchain contract b) Legal contract c) Smart contract d) Business contract
makefileCopy code**Answer: c) Smart contract**
12. What is the Ethereum Wallet primarily used for? a) Storing physical currency b) Mining cryptocurrency c) Deploying smart contracts d) Interacting with decentralized applications
csharpCopy code**Answer: d) Interacting with decentralized applications**
13. What does a Truffle framework provide for Ethereum development? a) Virtual reality simulations b) User authentication c) Smart contract development and testing d) Social media integration
makefileCopy code**Answer: c) Smart contract development and testing**
14. Which consensus algorithm requires participants to prove ownership of a certain number of cryptocurrency units to create or validate blocks? a) Proof of Work (PoW) b) Proof of Stake (PoS) c) Proof of Concept (PoC) d) Proof of Authority (PoA)
javaCopy code**Answer: b) Proof of Stake (PoS)**
15. What does a Solidity smart contract define? a) Styles and layouts for web pages b) Business logic and rules for a blockchain application c) Physical assets on the blockchain d) Social media profiles
makefileCopy code**Answer: b) Business logic and rules for a blockchain application**
16. What is the role of a blockchain in ensuring data integrity? a) Data duplication b) Data immutability c) Data compression d) Data encryption
makefileCopy code**Answer: b) Data immutability**
17. Which tool is commonly used to deploy and manage smart contracts on Ethereum? a) Remix IDE b) Photoshop c) Microsoft Word d) Google Chrome
makefileCopy code**Answer: a) Remix IDE**
18. What is the purpose of the Ethereum CLI (Command Line Interface)? a) To play games b) To access social media c) To interact with the Ethereum blockchain d) To edit images
vbnetCopy code**Answer: c) To interact with the Ethereum blockchain**
19. Which term describes the process of validating transactions and adding them to the blockchain in exchange for rewards? a) Mining b) Gaming c) Socializing d) Browsing
makefileCopy code**Answer: a) Mining**
20. What role does cryptography play in blockchain technology? a) Creating colorful designs b) Ensuring data immutability c) Generating music d) Solving complex mathematical equations
kotlinCopy code**Answer: b) Ensuring data immutability**
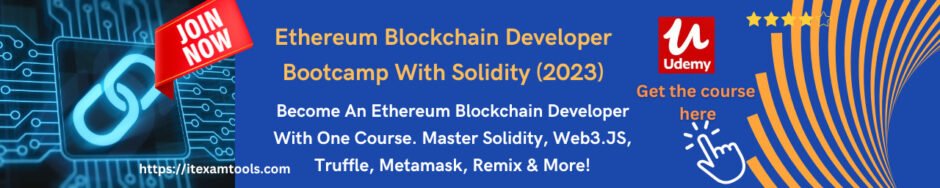