Data analysis with python and panda
Pandas is a powerful library in Python that is commonly used for data analysis and manipulation. It provides data structures such as Series (1-dimensional) and DataFrame (2-dimensional) that are similar to the data structures in R and can handle large amounts of data efficiently.
To use pandas, you first need to install it by running !pip install pandas
in a terminal or command prompt. Once you have it installed, you can import it into your Python script with the following line of code: import pandas as pd
.
Here are some common tasks that you can perform with pandas:
- Reading in data from a file (e.g. CSV, Excel, SQL) into a DataFrame
- Exploring and cleaning the data (e.g. checking for missing values, renaming columns, etc.)
- Filtering and selecting data using boolean indexing and query() method
- Grouping and aggregating data using groupby() method
- Merging and joining DataFrames
- Sorting and ordering data
- Visualising data using built-in plotting functions or Matplotlib library
Pandas is a powerful library that can handle many different types of data and it’s a great tool to have in your data analysis toolbox.
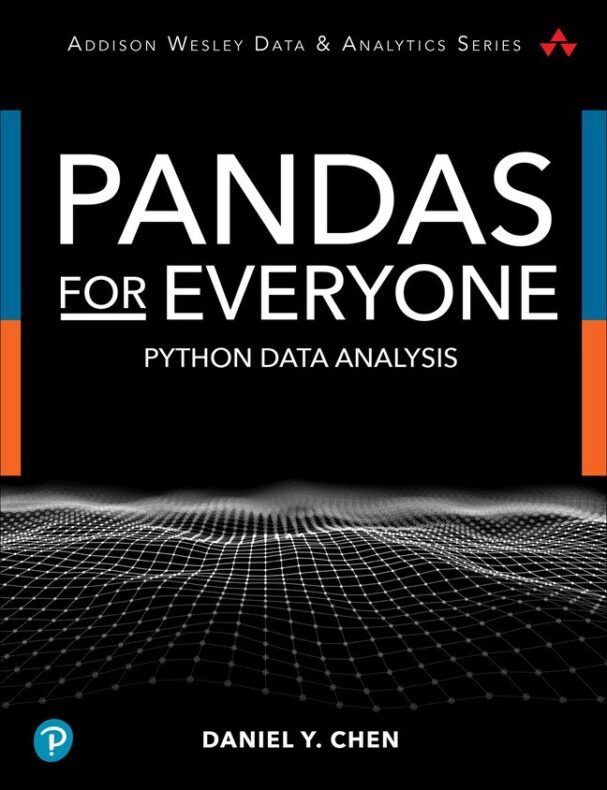
python with pandas example
This example assumes that you have a CSV file called ‘data.csv’ in the same directory as your script, and that the file contains columns called ‘age’ and ‘income’. The script reads in the data, views the first 5 rows, checks the data types and missing values, filters the data to only include rows where the ‘age’ column is greater than 30, groups the data by the ‘gender’ column and calculates the mean of each group, and finally, plots the data.
Here’s an example of how you can use pandas in Python to analyze a simple dataset:
import pandas as pd
read in data from a CSV file
data = pd.read_csv(‘data.csv’)
view the first 5 rows of the DataFrame
print(data.head())
check the data types of each column
print(data.dtypes)
check for missing values
print(data.isnull().sum())
select only the rows where the ‘age’ column is greater than 30
data = data[data[‘age’] > 30]
group the data by the ‘gender’ column and calculate the mean of each group
grouped_data = data.groupby(‘gender’).mean()
print(grouped_data)plot the data
data.plot(kind=’scatter’, x=’age’, y=’income’)
Keep in mind that this is a simple example and there are many other things you can do with python with pandas, such as merging and joining DataFrames, sorting and ordering data, and more.