Cisco OSPF Basic Configuration In this Cisco CCNA tutorial, you’ll learn how to do a basic configuration of OSPF on our Cisco routers. Scroll down for the video and also text…
Complete React Developer in 2023 (w/ Redux, Hooks, GraphQL)
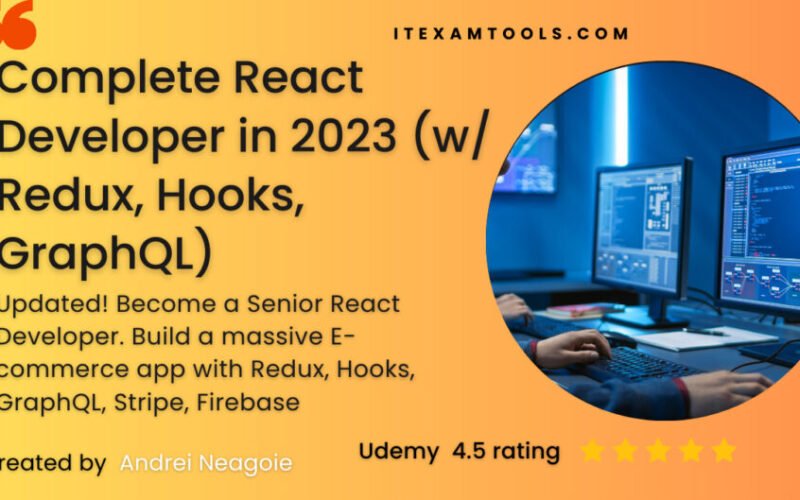
Updated! Become a Senior React Developer. Build a massive E-commerce app with Redux, Hooks, GraphQL, Stripe, Firebase
Supercharge Your IT Career with the Complete React Developer Course in 2023
Introduction: In today’s rapidly evolving tech landscape, staying ahead of the curve is crucial for IT students aiming to excel in their careers.
The Complete React Developer course on Udemy is a game-changing opportunity that empowers you to master the art of building modern, high-performance applications with React.
From learning the latest React features to gaining proficiency in state management, authentication, and even exploring GraphQL, this comprehensive course is designed to catapult your IT career to new heights. Let’s dive into the exciting learning journey that awaits you!
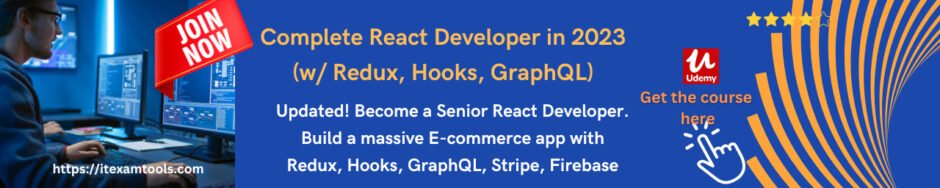
Look what you are going to learn…
- Build Enterprise-Level React Applications and Deploy with React 18: In a world where user expectations are sky-high, this course equips you with the skills to create impressive enterprise-level React applications. From concept to deployment, you’ll gain insights into React 18 and deploy your creations to production environments, demonstrating your ability to deliver top-notch solutions that align with industry standards.
- Learn to Build Reactive, Performant, Large Scale Applications: Aspiring IT professionals need to understand how to build applications that not only function well but also excel in terms of reactivity and performance. This course offers invaluable guidance, transforming you into a senior developer capable of architecting large-scale applications that impress both users and employers alike.
- Master the Latest Features in React: Staying current with the latest technologies is vital in the IT world. The course covers essential concepts such as Hooks, Context API, Suspense, React Lazy, and more. You’ll unlock the potential of these features, harnessing their power to create dynamic and efficient applications.
- Become the Top 10% ReactJS Developer: Distinguishing yourself in a competitive job market requires specialized expertise. By completing this course, you’ll position yourself as an elite ReactJS developer, gaining the skills needed to solve complex challenges and deliver results that set you apart.
- Utilize GraphQL as a React Developer: GraphQL has revolutionized the way data is fetched and managed. This course provides hands-on experience in integrating GraphQL with React, broadening your skill set and enabling you to work on cutting-edge projects.
- Harness the Power of Redux, Redux Thunk, and Redux Saga: State management is a cornerstone of modern web development. With a deep dive into Redux, Redux Thunk, and Redux Saga, you’ll grasp advanced state management techniques that streamline your application’s data flow.
- Compare Tradeoffs in State Management: Different projects demand different state management solutions. You’ll learn how to assess the tradeoffs between various state management approaches, ensuring you can make informed decisions to suit the unique needs of each project.
- Set Up Authentication and User Accounts: Security and user authentication are critical components of any application. By mastering authentication and user account management, you’ll enhance your ability to build secure, user-friendly experiences.
- Build Full Stack Applications with Firebase: Becoming proficient in full stack development opens doors to a wide array of opportunities. Through hands-on exercises, you’ll integrate Firebase into your applications, gaining insight into backend development and enhancing your skill set.
- Lead React Projects with Architecture Decisions: Strong leadership skills and architectural decision-making are key aspects of a successful IT career. This course empowers you to lead React projects confidently, making informed architecture choices and guiding your team to success.
- Master React Design Patterns: Elevate your coding skills by mastering React design patterns. These proven approaches will help you write cleaner, more maintainable code, contributing to your effectiveness as a developer.
- Explore CSS in JS with Styled-Components: Styling is an integral part of creating visually appealing applications. The course introduces you to CSS in JS using styled-components, enabling you to efficiently manage styles within your React applications.
- Navigate with React Router: Efficient navigation is crucial for user-friendly applications. By learning React Router, you’ll gain the skills needed to create smooth, intuitive navigation experiences that keep users engaged.
- Transform Apps into Progressive Web Apps (PWAs): As mobile usage continues to rise, developing Progressive Web Apps is a valuable skill. You’ll discover how to convert your applications into PWAs, providing users with seamless experiences across devices.
- Ensure Quality with Testing using Jest, Enzyme, and Snapshot Testing: Robust testing practices are essential for maintaining code quality. This course equips you with the knowledge to test your applications effectively using tools like Jest and Enzyme.
- Handle Online Payments with Stripe API: Monetizing applications is a vital aspect of software development. With insights into integrating the Stripe API, you’ll be prepared to handle online payments securely and efficiently.
- Embrace the Latest JavaScript Standards: A successful IT career hinges on writing clean, up-to-date code. By mastering the latest JavaScript standards (ES6 to ES11), you’ll ensure your codebase remains current and maintainable.
Conclusion: In the dynamic realm of IT, acquiring comprehensive skills is imperative for a successful career journey.
The Complete React Developer course on Udemy is a comprehensive package that provides you with all the tools, knowledge, and hands-on experience needed to excel as a React developer.
From mastering React’s latest features to becoming proficient in state management, authentication, and architecture decisions, this course empowers you to take your IT career to the next level.
By enrolling today, you’re investing in a brighter, more prosperous future as a top-tier ReactJS developer. Don’t miss out on this opportunity—seize it now and embark on your journey toward IT excellence!
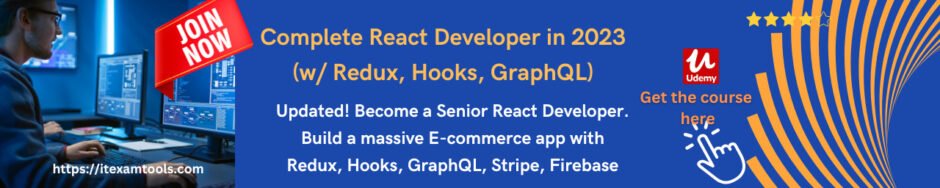
incorporating relevant code examples can help illustrate the practical aspects of the course content. Below,
I’ve included some code snippets that demonstrate key concepts covered in the Complete React Developer course:
1. Building a React Component with Hooks:
jsximport React, { useState, useEffect } from 'react'; const Counter = () => { const [count, setCount] = useState(0); useEffect(() => { document.title = `Count: ${count}`; }, [count]); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); }; export default Counter;
2. Using React Router for Navigation:
jsximport React from 'react'; import { BrowserRouter as Router, Route, Link } from 'react-router-dom'; const Home = () => <h1>Home Page</h1>; const About = () => <h1>About Us</h1>; const App = () => { return ( <Router> <nav> <ul> <li><Link to="/">Home</Link></li> <li><Link to="/about">About</Link></li> </ul> </nav> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Router> ); }; export default App;
3. Integrating Redux for State Management:
jsxmport React from 'react'; import { createStore } from 'redux'; import { Provider, connect } from 'react-redux'; // Redux actions and reducers const increment = () => ({ type: 'INCREMENT' }); const counterReducer = (state = 0, action) => { switch (action.type) { case 'INCREMENT': return state + 1; default: return state; } }; const store = createStore(counterReducer); // React component const Counter = ({ count, increment }) => ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); const mapStateToProps = state => ({ count: state }); const mapDispatchToProps = { inc
rement }; const ConnectedCounter = connect(mapStateToProps, mapDispatchToProps)(Counter); const App = () => ( <Provider store={store}> <ConnectedCounter /> </Provider> ); export default App;
4. Fetching Data with GraphQL:
jsxmport React from 'react';
import { useQuery } from '@apollo/client';
import { GET_USER } from './queries';
const UserProfile = ({ userId }) => {
const { loading, error, data } = useQuery(GET_USER, {
variables: { id: userId }
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const user = data.user;
return (
<div>
<h1>{user.name}</h1>
<p>Email: {user.email}</p>
</div>
);
};
export default UserProfile;
These code examples offer a glimpse into the practical aspects of the Complete React Developer course, showcasing the application of various concepts such as React Hooks, React Router, Redux state management, and GraphQL integration. By enrolling in the course, you’ll gain a deeper understanding of these topics and more, enabling you to build powerful, real-world applications that are essential for your IT career growth.
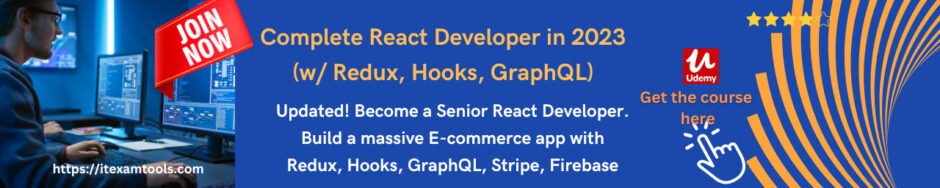
Here are 20 multiple-choice questions with answers related to the topics covered in the “Complete React Developer in 2023” course:
1. What is the purpose of React Hooks? a) Styling components b) Managing state and side effects c) Handling authentication d) Implementing routing
Answer: b) Managing state and side effects
2. Which React feature allows you to render components lazily to improve performance? a) React Router b) React Lazy c) React Suspense d) React Cache
Answer: b) React Lazy
3. Redux is a state management library that helps manage global state in your application. What is the main purpose of Redux Thunk? a) Handling asynchronous actions b) Creating reusable components c) Styling components d) Implementing routing
Answer: a) Handling asynchronous actions
4. What does GraphQL provide for fetching data from the server? a) A routing system b) A database management system c) A query language for APIs d) A state management solution
Answer: c) A query language for APIs
5. Which React feature is used to manage navigation and routing within a single-page application? a) React Hooks b) React Router c) React Lazy d) React Context
Answer: b) React Router
6. In Redux, what is the purpose of a reducer? a) Handling side effects b) Managing state changes c) Styling components d) Managing authentication
Answer: b) Managing state changes
7. What is the primary role of Firebase in a full stack application? a) Handling user authentication b) Handling routing and navigation c) Managing global state d) Implementing styling
Answer: a) Handling user authentication
8. What does React Context API provide? a) A way to manage global state b) A way to fetch data from APIs c) A way to handle user authentication d) A way to handle routing
Answer: a) A way to manage global state
9. What is the purpose of Progressive Web Apps (PWAs)? a) To create complex animations b) To enhance user experience on mobile devices c) To manage global state d) To handle user authentication
Answer: b) To enhance user experience on mobile devices
10. Which testing library is commonly used for testing React applications? a) Mocha b) Enzyme c) Jasmine d) Jest
Answer: d) Jest
11. What is the primary purpose of styled-components in React development? a) Managing global state b) Handling asynchronous actions c) Styling React components d) Handling routing and navigation
Answer: c) Styling React components
12. Which JavaScript feature allows you to iterate over elements in an array using concise syntax? a) Loops b) Map function c) For…of loop d) forEach function
Answer: c) For…of loop
13. What does Redux Saga provide in Redux-based applications? a) A way to manage routing b) A middleware for handling asynchronous actions c) A query language for APIs d) A state management solution
Answer: b) A middleware for handling asynchronous actions
14. What is the purpose of the “useState” hook in React? a) To manage asynchronous actions b) To manage global state c) To manage component state d) To manage routing and navigation
Answer: c) To manage component state
15. What is the role of “React Suspense” in React applications? a) Handling user authentication b) Managing global state c) Handling asynchronous rendering and data fetching d) Managing routing and navigation
Answer: c) Handling asynchronous rendering and data fetching
16. What is the main purpose of Redux in React applications? a) Handling routing and navigation b) Managing global state c) Styling components d) Handling user authentication
Answer: b) Managing global state
17. Which of the following is used for testing React components and checking how they render over time? a) Snapshot testing b) Unit testing c) Integration testing d) Performance testing
Answer: a) Snapshot testing
18. What is the primary function of the “connect” function from the “react-redux” library? a) Styling components b) Handling user authentication c) Connecting React components to the Redux store d) Managing routing and navigation
Answer: c) Connecting React components to the Redux store
19. What does “React Lazy” help you achieve in your application? a) Efficient code splitting and lazy loading of components b) Global state management c) Handling asynchronous actions d) Styling components
Answer: a) Efficient code splitting and lazy loading of components
20. What is the primary goal of using GraphQL in a React application? a) Handling user authentication b) Simplifying component structure c) Efficiently fetching and managing data from APIs d) Managing routing and navigation
Answer: c) Efficiently fetching and managing data from APIs
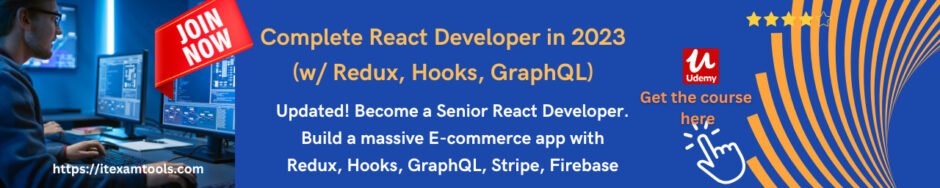