Introduction In the modern era of cloud computing, software architecture plays a crucial role in enabling organizations to leverage the full potential of the cloud. With the increasing adoption of…
Best 20 React Coding Questions with Answers for Coding Interview
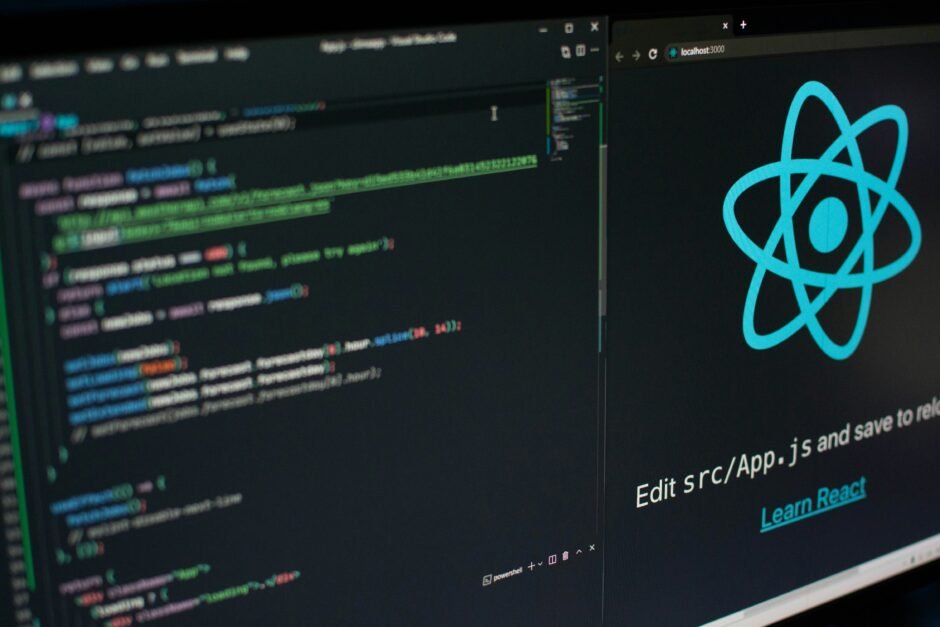
In this blog post, we will explore 20 common React coding questions that often come up during coding interviews.
Each question will be accompanied by a detailed answer and, where appropriate, code examples to help you prepare for your next interview. you should surely learn these React Coding Questions.
Learn about React, its key features, JSX, creating React components, handling events, React lifecycle methods, passing data between components, optimizing performance, and handling forms in React.
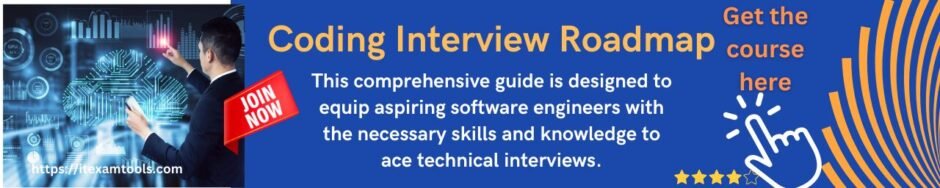
Introduction to React Coding Questions with Answers
React is a popular JavaScript library used for building user interfaces. As a React developer, it is essential to have a strong understanding of the core concepts and best practices.
In this blog post, we will explore 20 common React coding questions that often come up during coding interviews.
Each question will be accompanied by a detailed answer and, where appropriate, code examples to help you prepare for your next interview.
1. What is React?
React is a JavaScript library developed by Facebook for building user interfaces.
It allows developers to create reusable UI components and efficiently update the UI when the underlying data changes.
React follows a component-based architecture and uses a virtual DOM for efficient rendering.
Answer:
React is a declarative, efficient, and flexible JavaScript library for building user interfaces.
It uses a virtual DOM to efficiently update only the necessary parts of the UI when the underlying data changes. React components are reusable and can be composed to build complex UIs.
2. What are the key features of React?
Answer:
- Declarative: React allows developers to describe the desired UI state, and it automatically updates the UI when the underlying data changes.
- Component-Based: React follows a component-based architecture, where UIs are built by composing reusable components.
- Virtual DOM: React uses a virtual DOM to efficiently update the UI by comparing the current and previous states.
- Unidirectional Data Flow: React enforces a unidirectional data flow, making it easier to understand and debug the application.
- JSX: React uses JSX, a syntax extension that allows developers to write HTML-like code within JavaScript.
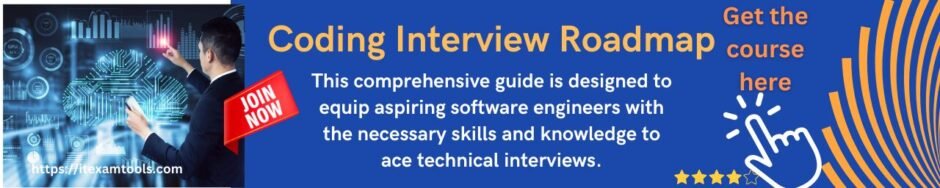
3. What is JSX?
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. It is used in React to define the structure and appearance of UI components.
Answer:
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript.
It provides a concise and familiar syntax for defining the structure and appearance of UI components in React. JSX code is transpiled to regular JavaScript code before being executed in the browser.
4. How do you create a React component?
In React, components can be created as either functional components or class components.
Answer:
A functional component is a JavaScript function that returns JSX code. Here’s an example:
function MyComponent() {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a functional component.</p>
</div>
);
}
A class component is a JavaScript class that extends the React.Component class. It requires a render method that returns JSX code. Here’s an example:
class MyComponent extends React.Component {
render() {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a class component.</p>
</div>
);
}
}
5. What is the difference between state and props in React?
In React, both state and props are used to manage data within a component, but they have different purposes and behaviors.
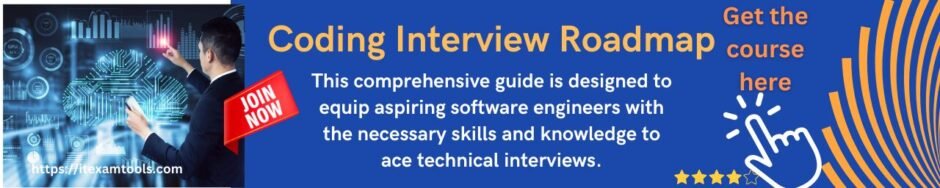
Answer:
State: State is used to manage internal data within a component. It is mutable and can be updated using the setState()
method. When the state changes, React re-renders the component to reflect the new state.
Props: Props are used to pass data from a parent component to a child component.
They are immutable and cannot be modified by the child component. Props are passed as attributes to the child component and can be accessed using this.props
.
6. How do you handle events in React?
In React, event handling is similar to handling events in HTML. You can attach event handlers to JSX elements using the onEvent
syntax.
Answer:
To handle events in React, you can define event handler methods within your component and then attach them to JSX elements. Here’s an example of handling a button click event:
class MyComponent extends React.Component {
handleClick() {
console.log('Button clicked!');
}
render() {
return (
<button onClick={this.handleClick}>Click Me</button>
);
}
}
In this example, the handleClick()
method will be called when the button is clicked, and it will log a message to the console.
7. What are React lifecycle methods?
React provides a set of lifecycle methods that allow you to perform actions at specific points in the lifecycle of a component.
These methods can be overridden in class components to add custom behavior.
Answer:
Here are some of the commonly used React lifecycle methods:
- componentDidMount: This method is called after the component has been rendered to the DOM. It is commonly used for fetching data from an API or setting up event listeners.
- componentDidUpdate: This method is called after the component has been updated. It is commonly used for updating the component’s state or interacting with the updated DOM.
- componentWillUnmount: This method is called before the component is removed from the DOM. It is commonly used for cleaning up resources or canceling ongoing network requests.
8. How do you pass data between components in React?
In React, data can be passed between components using props. Props allow you to pass data from a parent component to a child component.
Answer:
To pass data between components, you can pass props from the parent component to the child component. Here’s an example:
class ParentComponent extends React.Component {
render() {
const data = 'Hello, child component!';
return (
<ChildComponent data={data} />
);
}
}
class ChildComponent extends React.Component {
render() {
return (
<p>{this.props.data}</p>
);
}
}
In this example, the data
prop is passed from the ParentComponent
to the ChildComponent
and rendered as a paragraph.
9. How can you optimize performance in React?
Optimizing performance in React is crucial for delivering a smooth user experience. Here are some techniques to improve performance:
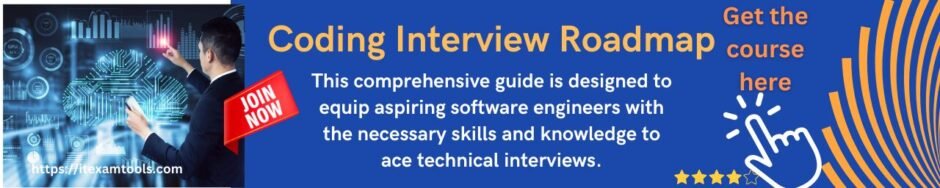
Answer:
- Use PureComponent: PureComponent is a base class provided by React that implements a shallow comparison of props and state. It prevents unnecessary re-renders when the data hasn’t changed.
- Use React.memo: React.memo is a higher-order component that memoizes the result of a functional component. It can be used to prevent unnecessary re-renders of functional components.
- Use shouldComponentUpdate: By implementing the
shouldComponentUpdate()
method in class components, you can control when the component should re-render. It allows you to optimize performance by preventing unnecessary re-renders. - Use key prop: When rendering lists of components, make sure to provide a unique key prop for each item. This helps React efficiently update the list without re-rendering all the items.
- Use code splitting: Code splitting is a technique that allows you to split your code into smaller chunks and load them on-demand. It can significantly improve the initial load time of your application.
10. How do you handle forms in React?
Handling forms in React involves capturing user input and updating the component’s state accordingly.
Answer:
To handle forms in React, you can use the controlled component approach.
In this approach, the component’s state is used to store the form data, and the input values are set by the state. Here’s an example:
class MyForm extends React.Component {
constructor(props) {
super(props);
this.state = {
username: '',
password: ''
};
}
handleChange(event) {
this.setState({ [event.target.name]: event.target.value });
}
handleSubmit(event) {
event.preventDefault();
// Perform form submission logic here
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<input
type="text"
name="username"
value={this.state.username}
onChange={this.handleChange}
/>
<input
type="password"
name="password"
value={this.state.password}
onChange={this.handleChange}
/>
<button type="submit">Submit</button>
</form>
);
}
}
In this example, the handleChange()
method updates the component’s state whenever the input values change, and the handleSubmit()
method handles the form submission logic.
Conclusion
In this blog post, we have covered 10 common React coding questions that you may encounter during a coding interview.
By understanding and practicing these React Coding Questions, you will be better prepared to showcase your React skills and knowledge.
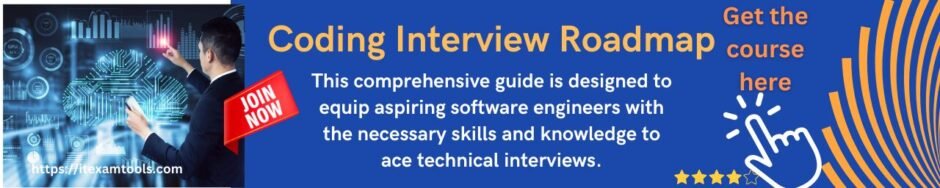
Remember to focus on the core concepts of React, such as components, state, props, and event handling, as these are fundamental to building robust and efficient React applications.
Good luck with your coding interviews! by learning these React Coding Questions
https://itexamsusa.blogspot.com/2023/12/mastering-matlab-programming-for.html
https://itexamsusa.blogspot.com/2023/12/monolith-vs-microservices-which-one-is.html
https://itexamsusa.blogspot.com/2023/12/publicprivate-keypairs-and-generating.html
https://itexamsusa.blogspot.com/2023/10/exam-dp-203-data-engineering-on.html
https://itexamsusa.blogspot.com/2023/10/ccnp-enterprise-advanced-routing-enarsi.html
https://itexamsusa.blogspot.com/2023/10/red-hat-certified-engineerrhce-ex294.html
https://itexamsusa.blogspot.com/2023/09/github-actions-to-auto-build-your.html