A Complete Steps Guide for Beginners interested in learning HTML CSS JavaScript to Build Interactive Web HTML CSS JavaScript Course For Modern Web Developers Description In this course, we will…
20+ Free JavaScript Projects to Explore and Build

20+ Free JavaScript Projects to Explore and Build
JavaScript: The Most Popular Programming Language
According to the 2023 Stack Overflow Developer Survey, JavaScript has maintained its position as the most widely used programming language for 11 consecutive years, with 66% of developers using it extensively. If you’re aspiring to become a web developer—whether in front-end, back-end, or full-stack development—JavaScript is one of the most valuable programming languages to master. A practical way to start learning is by working on beginner-friendly JavaScript projects.
Understanding Vanilla JavaScript
Vanilla JavaScript, often referred to as plain JavaScript (without any frameworks or libraries), is a cornerstone of coding and web development. Most websites are built using a combination of HTML, CSS, vanilla JavaScript, and API integrations. Familiarizing yourself with JavaScript fundamentals is essential, and the best way to solidify your knowledge is by building hands-on JavaScript practice projects.
The Importance of Practice Projects
Practical experience is invaluable for honing JavaScript skills. Thousands of students in the Break into Tech front-end development program have worked on exciting beginner-friendly projects to build their expertise. These projects not only help learners practice coding but also contribute to their portfolios, showcasing their abilities to potential employers.
Many of our successful students who secured jobs at leading companies such as GoDaddy, Toast, Asics Digital, 1Password, Figure, and Apple had one thing in common: a solid foundation in JavaScript, impressive portfolios, and hands-on practice through JavaScript projects.
Start Building Your Portfolio with Beginner Projects
To help you practice and enhance your front-end development skills, we’ve compiled a list of over 20 beginner-friendly JavaScript projects. These open-source projects include access to source code, which you can use as a reference or inspiration. Whether you’re exploring JavaScript for the first time or strengthening your existing skills, these projects are a great place to start.
Simply find a project that interests you and dive in! The source code for each project is linked on its homepage, allowing you to experiment, learn, and grow as a developer.
Tips for Learning Through JavaScript Projects
Lisa Savoie, a JavaScript instructor at Skillcrush, offers valuable advice for working on practice projects. She recommends:
- Reading source code out loud to better understand its structure and logic.
- Identifying features that you can integrate into your own projects.
- Retyping the code to develop muscle memory for writing functions, variables, and loops.
She adds, “If you encounter unfamiliar methods, Google them to deepen your understanding. Don’t be afraid to break the code and fix it—breaking things can be a fun and educational experience!” 😄
Ready to Begin?
Explore our curated list of 20+ JavaScript beginner projects and start building your skills today. Whether you’re reading code, experimenting with new features, or creating your own projects, every step you take brings you closer to becoming a proficient JavaScript developer.
Lets first see some basic code examples for the JavaScript before we see actual projects
1. Hello World
javascriptCopy codeconsole.log("Hello, World!");
Explanation: Prints “Hello, World!” to the browser console. A great way to test if your JavaScript is working.
2. Variables
javascriptCopy codelet name = "Alice";
const age = 25;
var isStudent = true;
Explanation:
let
: Declares a variable that can be updated.const
: Declares a constant that cannot change.var
: An older way to declare variables (less commonly used now).
3. Simple Function
javascriptCopy codefunction greet(name) {
return `Hello, ${name}!`;
}
console.log(greet("Alice"));
Explanation: Defines a function greet
that takes a name as input and returns a personalized greeting.
4. If-Else Conditional
javascriptCopy codelet age = 18;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
Explanation: Checks if age
is 18 or older and logs a message accordingly.
5. For Loop
javascriptCopy codefor (let i = 1; i <= 5; i++) {
console.log(i);
}
Explanation: Loops from 1 to 5, printing each number to the console.
6. Array
javascriptCopy codelet fruits = ["apple", "banana", "cherry"];
console.log(fruits[1]); // Outputs: banana
Explanation: Creates an array and accesses its second element (index 1).
7. Object
javascriptCopy codelet person = {
name: "Alice",
age: 25,
isStudent: true
};
console.log(person.name); // Outputs: Alice
Explanation: Creates an object with properties and accesses the name
property.
8. Event Listener
javascriptCopy codedocument.querySelector("button").addEventListener("click", () => {
alert("Button clicked!");
});
Explanation: Attaches a click event listener to a button, displaying an alert when clicked.
9. Set Timeout
javascriptCopy codesetTimeout(() => {
console.log("This appears after 2 seconds.");
}, 2000);
Explanation: Executes a function after 2 seconds (2000 milliseconds).
10. Random Number
javascriptCopy codelet randomNumber = Math.random() * 10;
console.log(Math.floor(randomNumber));
Explanation: Generates a random number between 0 and 10, then rounds it down to the nearest integer.
Here is the list of the JavaScript projects for the beginners we are going to explore!
- Mouseover Element
- Clock
- Magic 8 Ball
- Build a To-Do List
- Epic Mix Playlist
- Pet Rescue
- Drum Kit
- Speech Detection
- Sticky Navigation
- Geolocation
- Movie App
- Name Tags
- Intermediate JavaScript Projects
- Tone.js
- Election Map
- Login Authentication
- Guess the Word
- Terminalizer
- Chat App
- Tic Tac Toe Game
- Hotel Booking App
- Advanced JavaScript Project
- Maze Game
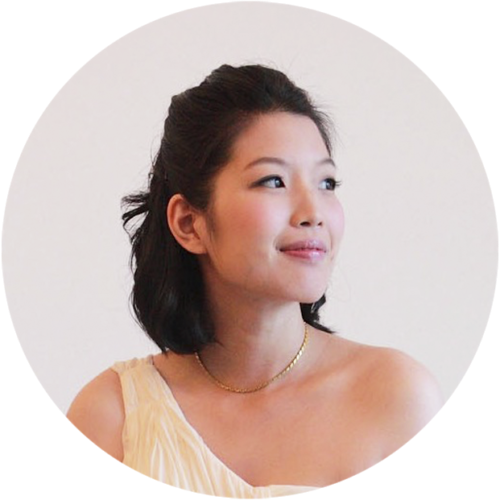
Justina Hwang
Justina Hwang is Content Marketing Manager at Skillcrush, and has been covering tech education for over three years. She holds a PhD from Brown University. Justina spends her free time with her mildly needy (but very adorable) cat.
Agile project management Artificial Intelligence aws blockchain cloud computing coding interview coding interviews Collaboration Coursera css cybersecurity cyber threats data analysis data breaches data science data visualization devops django docker excel flask hacking html It Certification java javascript ketan kk Kubernetes machine learning mongoDB Network & Security network protocol nodejs online courses online learning Operating Systems Other It & Software pen testing python Software Engineering Terraform Udemy courses VLAN web development